Domob DBox SDK
关于多宝屋
- 请参见 About DBox
必要条件
Xcode 6
或更高版本iOS 5.0
或更高版本
获取SDK
请发送邮件到 support-dbox@domob.cn 获取最新的 iOS DBox SDK 发行包。
配置通用参数
1 编译参数:多宝屋 SDK 同时支持 ARC/Non-ARC 编译选项。主要是在代码中使用 DOARCMarcos 来加以 控制。
- (void)doEntryClick
{
if (dboxManager) {
[dboxManager sendAdEntryClickReport];
}
}
- (void)doEntryImpression
{
if (dboxManager) {
[dboxManager sendAdEntryImpressionReport];
}
}
- (void)dealloc
{
DO_SAFE_ARC_RELEASE(_adChannelInfos);
DO_SAFE_ARC_RELEASE(_adCreativeInfos);
DO_SAFE_ARC_RELEASE(_adControlInfo);
DO_SAFE_ARC_RELEASE(dboxManager);
DO_SAFE_ARC_RELEASE(preloadButton);
DO_SAFE_ARC_SUPER_DEALLOC();
}
2 链接多宝屋 DBoxSDK framework
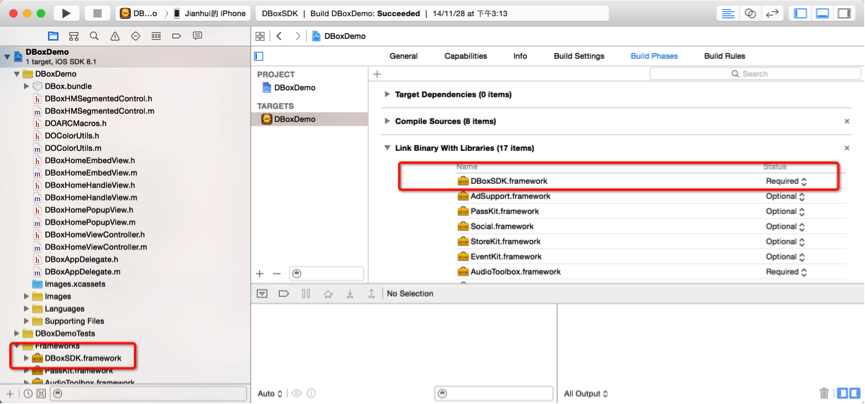
目录结构和文件组织
iOS 多宝屋SDK发行版本目录结构如下
-
SDK 文件
-
SDK framework:DBoxSDK.framework,包含多种架构,如,armv7, armv7s, arm64, i368, x86-64 等; Framework 中也包含了,
-
头文件:Header
-
库:DBoxSDK
-
-
UI 图片资源包,DBox.bundle
-
开发者文档:DBoxSDKDeveloperGuide.pdf
-
-
SDK Demo项目
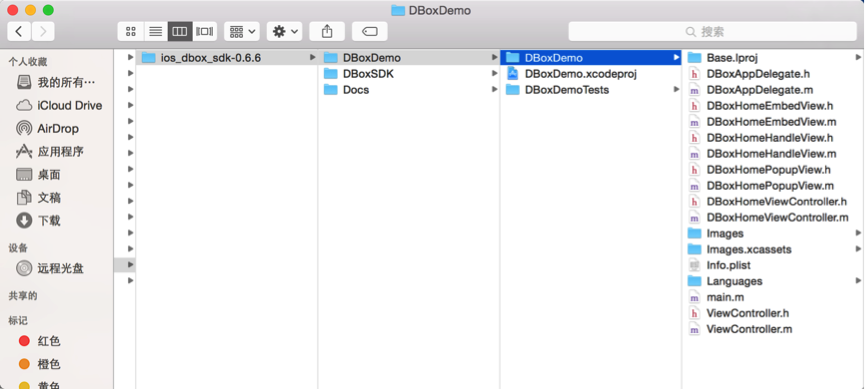
-
SDK 重要头文件介绍
-
数据相关的头文件
- DBoxManager.h
- DBoxManager.h
-
工具相关的头文件
- DOARCMacros 定义了一组 ARC/Non-ARC 相关的宏,使用这些宏而无需关注项目是 ARC,还是 Non-ARC。
- DOARCMacros 定义了一组 ARC/Non-ARC 相关的宏,使用这些宏而无需关注项目是 ARC,还是 Non-ARC。
-
SDK的使用方式和步骤
开发者可以使用 SDK 提供的模版 UI 和 Control 来展示多宝屋广告,可以节省一些界面开发的时间。效果如下,
入口 | 主界面 |
---|---|
![]() |
![]() |
上面是 Demo 应用对模版的使用效果,当点击“加载数据”后,多宝屋模版入口即可弹出,并按照服务器后台设置的模式(如,平铺、卡片等)展现广告。 模版的使用由一个重要的类DBoxManager提供接口,目前DBoxManager提供两种使用方式:
方式一:预加载模式
下面就Demo为例,详细说明模版的使用步骤
1 准备好申请的开发者ID(Publisher ID)和多宝屋的广告位 ID(Placement ID),如,Demo中是将此ID放入在 AppDelegate中,
#import <UIKit/UIKit.h>
#import "DOARCMacros.h"
#define DBOX_PUBLISHER_ID_ONLINE @"56OJw+rouN8xdhPaW9"
#define DBOX_PLACEMENT_ID_ONLINE @"16TLuFnvAp2p4NU06al1hNgi"
@class DBoxHomeViewController;
@interface DBoxAppDelegate : UIResponder <UIApplicationDelegate>
@property (nonatomic, DO_STRONG) UIWindow *window;
@property (nonatomic, DO_STRONG) UINavigationController *navController;
@property (nonatomic, DO_STRONG) DBoxHomeViewController *rootController;
@property (nonatomic, DO_STRONG) NSString *publisherId;
@property (nonatomic, DO_STRONG) NSString *placementId;
@end
@implementation DBoxAppDelegate
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
[[UIApplication sharedApplication] setStatusBarHidden:NO withAnimation:UIStatusBarAnimationSlide];
[[UIApplication sharedApplication] setStatusBarStyle:UIStatusBarStyleLightContent];
self.rootController = DO_SAFE_ARC_AUTORELEASE([[DBoxHomeViewController alloc] init]);
self.navController = DO_SAFE_ARC_AUTORELEASE([[UINavigationController alloc] initWithRootViewController:self.rootController]);
self.window = DO_SAFE_ARC_AUTORELEASE([[UIWindow alloc] initWithFrame:[[UIScreen mainScreen] bounds]]);
[self.window setRootViewController:self.navController];
// Override point for customization after application launch.
[self.window setBackgroundColor:[UIColor whiteColor]];
[self.window makeKeyAndVisible];
[self setPublisherId:DBOX_PUBLISHER_ID_ONLINE];
[self setPlacementId:DBOX_PLACEMENT_ID_ONLINE];
return YES;
}
2 声明 DBoxManager,并监听DBoxManagerDelegate。
@interface DBoxHomePopupView () <UIGestureRecognizerDelegate, DBoxManagerDelegate> {
DBoxManager *dboxManager;
UIButton *preloadButton;
UIButton *bannerRatioButton;
}
3 确定何时请求网络数据。可以在你App界面的初始化阶段获取,也可以在某个按钮点击后获取。比如,Demo中是点击“加载数据”按钮后获取。 此时,我们初始化 dboxManager实例,并设置dboxManager的delegate,然后调用preloadData向服务器请求数据。
- (void)handlePreloadButtonTapped:(id)sender
{
DBoxAppDelegate *appDelegate = (DBoxAppDelegate *)[[UIApplication sharedApplication] delegate];
dboxManager = [[DBoxManager alloc] initWithPublisherId:appDelegate.publisherId placementId:appDelegate.placementId rootViewController:appDelegate.rootController];
[dboxManager setDelegate:self];
// [dboxManager setNavigationBarTintColor:[UIColor redColor]];
// [dboxManager setNavigationBarTintColorHexString:@"5abc21"];
// [dboxManager setNavigationBarBackgroundImageNamed:@"bg_nav"];
[dboxManager preloadData];
[self doEntryClick];
}
4 在点击入口按钮后,发送入口点击报告,
- (void)doEntryClick
{
if (dboxManager) {
[dboxManager sendAdEntryClickReport];
}
}
5 在数据获取成功后,展示模版入口界面,
#pragma mark -
#pragma mark - DBoxdboxManagerDelegate
- (void)dboxDidFinishLoad
{
[dboxManager presentEntryViewController];
[self doEntryImpression];
}
获取获取成功后,此时所有的数据已经预加载完毕,那么我们可以根据需要,
立刻调用
;也可以在其它方便的时候调用
[dboxManager presentEntryController]来展示入口界面。
6 在入口界面展示后,发送入口展现报告,
- (void)doEntryImpression
{
if (dboxManager) {
[dboxManager sendAdEntryImpressionReport];
}
}
7 DBoxManager 也提供了完备的回调机制,将重要的事件通知给 App,App可以根据需要来进行应对,包括,
@protocol DBoxManagerDelegate <NSObject>
@optional
//
// 多宝屋数据请求完毕
//
- (void)dboxDidFinishLoad;
//
// 多宝屋数据请求失败
// @param error 错误数据
//
- (void)dboxDidFailedLoadWithError:(NSError *)error;
//
// 多宝屋界面展现
//
- (void)dboxDidAdViewPresented;
//
// 多宝屋界面退出
//
- (void)dboxDidAdViewDismissed;
//
// 多宝屋界面进入后台
//
- (void)dboxDidAppEnterBackground;
@end
如,Demo中对delegate的处理,
#pragma mark -
#pragma mark - DBoxdboxManagerDelegate
- (void)dboxDidFinishLoad
{
[dboxManager presentEntryViewController];
[self doEntryImpression];
}
- (void)dboxDidFailedLoadWithError:(NSError *)error
{
NSLog(@"Failed to load:%@", error);
}
- (void)dboxDidAdViewPresented
{
NSLog(@"Ad view presented");
}
- (void)dboxDidAdViewDismissed
{
NSLog(@"Ad view dismissed");
}
- (void)dboxDidAppEnterBackground
{
NSLog(@"App enter background");
}
方式二:直接展示模式
1 准备好申请的开发者ID(Publisher ID)和多宝屋的广告位 ID(Placement ID),如,Demo中是将此ID放入在 AppDelegate中,
#import <UIKit/UIKit.h>
#import "DOARCMacros.h"
#define DBOX_PUBLISHER_ID_ONLINE @"56OJw+rouN8xdhPaW9"
#define DBOX_PLACEMENT_ID_ONLINE @"16TLuFnvAp2p4NU06al1hNgi"
@class DBoxHomeViewController;
@interface DBoxAppDelegate : UIResponder <UIApplicationDelegate>
@property (nonatomic, DO_STRONG) UIWindow *window;
@property (nonatomic, DO_STRONG) UINavigationController *navController;
@property (nonatomic, DO_STRONG) DBoxHomeViewController *rootController;
@property (nonatomic, DO_STRONG) NSString *publisherId;
@property (nonatomic, DO_STRONG) NSString *placementId;
@end
@implementation DBoxAppDelegate
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
[[UIApplication sharedApplication] setStatusBarHidden:NO withAnimation:UIStatusBarAnimationSlide];
[[UIApplication sharedApplication] setStatusBarStyle:UIStatusBarStyleLightContent];
self.rootController = DO_SAFE_ARC_AUTORELEASE([[DBoxHomeViewController alloc] init]);
self.navController = DO_SAFE_ARC_AUTORELEASE([[UINavigationController alloc] initWithRootViewController:self.rootController]);
self.window = DO_SAFE_ARC_AUTORELEASE([[UIWindow alloc] initWithFrame:[[UIScreen mainScreen] bounds]]);
[self.window setRootViewController:self.navController];
// Override point for customization after application launch.
[self.window setBackgroundColor:[UIColor whiteColor]];
[self.window makeKeyAndVisible];
[self setPublisherId:DBOX_PUBLISHER_ID_ONLINE];
[self setPlacementId:DBOX_PLACEMENT_ID_ONLINE];
return YES;
}
2 声明 DBoxManager,并监听DBoxManagerDelegate。
@interface DBoxHomePopupView () <UIGestureRecognizerDelegate, DBoxManagerDelegate> {
DBoxManager *dboxManager;
UIButton *preloadButton;
UIButton *bannerRatioButton;
}
3 立即请求网络数据,并展示入口界面,
此时,我们调用initWithPublisherId:placementId:rootViewController初始化 dboxManager实例,并设置dboxManager的delegate。
- (void)handlePreloadButtonTapped:(id)sender
{
DBoxAppDelegate *appDelegate = (DBoxAppDelegate *)[[UIApplication sharedApplication] delegate];
dboxManager = [[DBoxManager alloc] initWithPublisherId:appDelegate.publisherId placementId:appDelegate.placementId rootViewController:appDelegate.rootController];
[dboxManager setDelegate:self];
// [dboxManager setNavigationBarTintColor:[UIColor redColor]];
// [dboxManager setNavigationBarTintColorHexString:@"5abc21"];
// [dboxManager setNavigationBarBackgroundImageNamed:@"bg_nav"];
[dboxManager presentEntryViewController];
[self doEntryClick];
}
4 在点击入口按钮后,发送入口点击报告,
- (void)doEntryClick
{
if (dboxManager) {
[dboxManager sendAdEntryClickReport];
}
}
5 在入口界面展示后,发送入口展现报告,
- (void)doEntryImpression
{
if (dboxManager) {
[dboxManager sendAdEntryImpressionReport];
}
}
备注
1 DBoxManager支持多个广告位,也就是说,我们可以用不同的广告位ID(Placement ID)来实例化多个DBoxManager;
2 通过后台设置,我们也可以使不同广告位ID的DBoxManager展示不同模式的入口界面,即平铺模式和卡片模式;
平铺模式(flat) | 卡片模式(card) |
---|---|
![]() |
![]() |
3 DBoxManager 也提供了三个接口供用户修改导航栏颜色,分别是,
//
// 设置导航栏颜色。
// @param barTintColor 颜色
//
- (void)setNavigationBarTintColor:(UIColor *)barTintColor;
//
// 设置导航栏颜色。
// @param barTintColorHexString 十六进制颜色字符串
//
- (void)setNavigationBarTintColorHexString:(NSString *)barTintColorHexString;
//
// 设置导航栏背景图。
// @param barBackgroundImageName 背景图的文件名
//
- (void)setNavigationBarBackgroundImageNamed:(NSString *)barBackgroundImageName;
红色导航栏 | 绿色导航栏 | |
---|---|---|
![]() |
![]() |
SDK模版嵌入方式
SDK 附带的demo展示了三种SDK模版嵌入方式,分别是:
1 固定&弹出 最常用也是最普通的方式,如,设定一个界面上的按钮作为多宝屋的入口,那么当点击某个按钮时,入口界面即弹出;
固定&弹出 | 主界面 | |
---|---|---|
![]() |
![]() |
2 小把手&弹出 为节省界面空间,也可以用一个侧边把手来作为入口,当点击后,弹出入口界面。
小把手&弹出 | 主界面 | |
---|---|---|
![]() |
![]() |
3 嵌入式 将多宝屋的界面融入您的app之中,使其自然成为app的一部分,
嵌入式 | 主界面 | |
---|---|---|
![]() |
![]() |
具体的使用请参见SDK所附带的demo程序。
注意事项
多宝屋 iOS SDK集成注意事项:
-
Publisher ID 和 Placement ID 一定要用媒体自己申请的,不要用多宝屋Demo中的默认 ID;
-
发送入口点击报告
-
发送入口展现报告:
请您在集成完毕后务必逐一核查以上各项。保证各项的正确性,以免影响收入。
审核注意事项
1 由于多宝屋 SDK 获取了 IDFA,那么在提交 App Store 审核时,请按照下面的截图选择 IDFA 相关的选项。
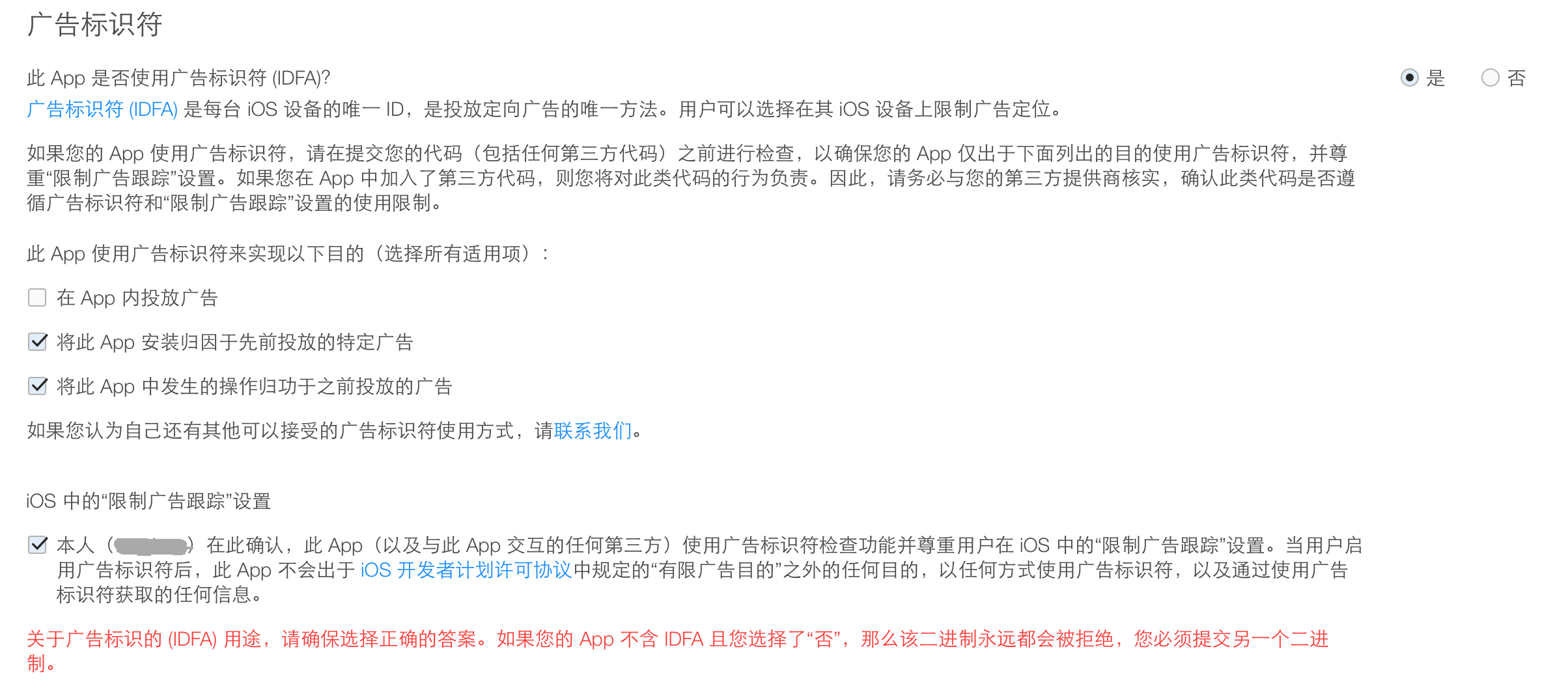
2 若以上选择仍然不能通过审核,
- 请按照下面的截图选择 IDFA 相关的选项
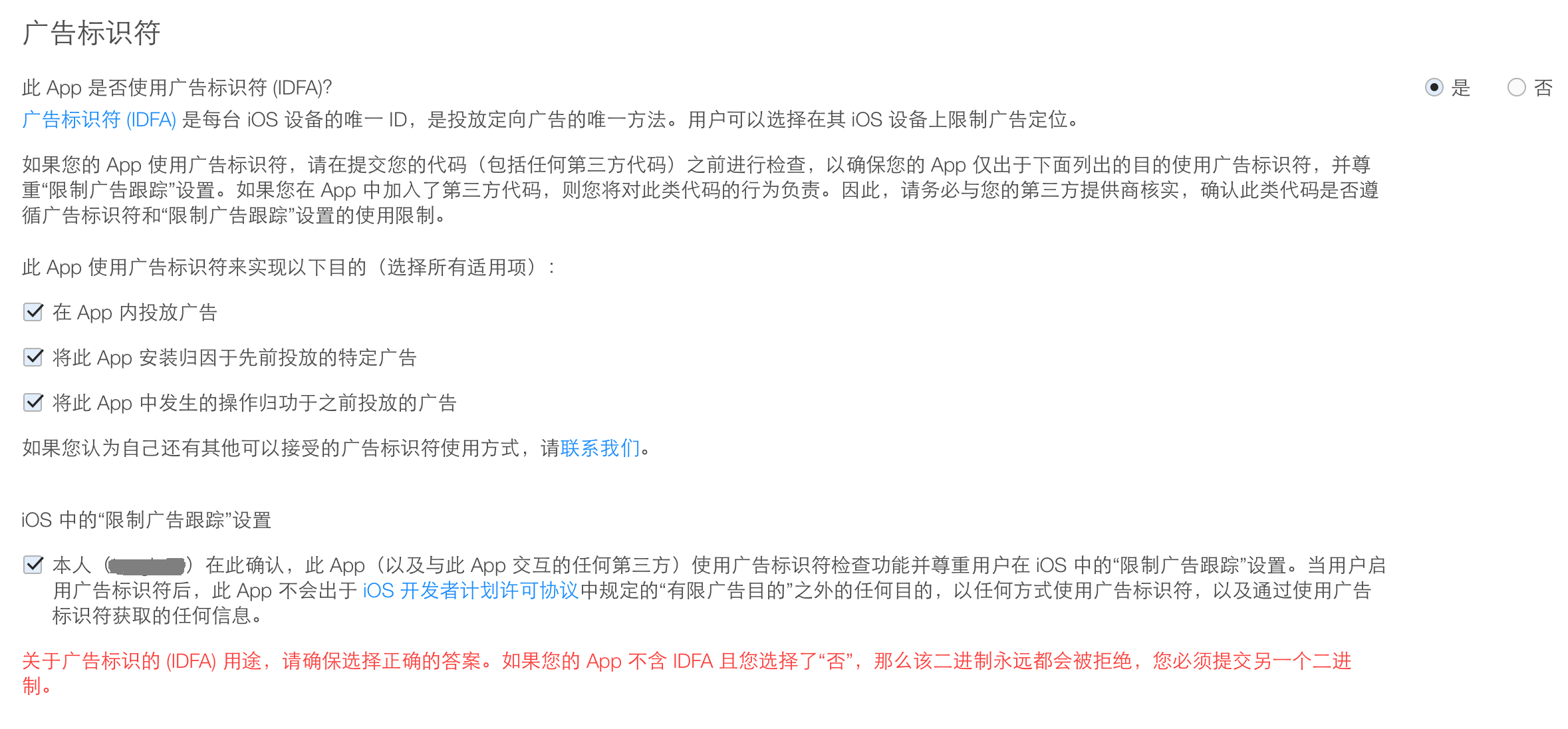
- 业务管理后台打开多宝屋的 banner 广告
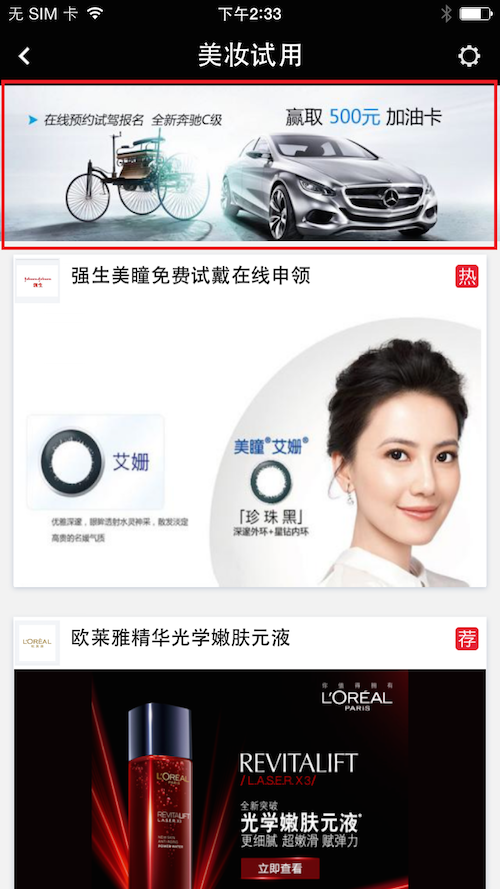
- 在审核界面的“备注”中,务必用英文或者中英文,详细说明找到多宝屋 banner 广告的步骤。